Button
The button is a control that reacts to pointer actions (and has some keyboard equivalents). It presents visual feedback in the form of a depressed state when the pointer is down.
A pointer-down to pointer release sequence is interpreted as a click; and this behaviour is configurable.
When determining if a button is pressed by the user, always use the Click
event instead of PointerPressed
. Click
is the high-level event specific to a Button
that indicates it has been pressed.
PointerPressed
is more a low-level input event: one that the Button
needs to handle internally to raise the Click
event. Since Button
handles PointerPressed
(sets IsHandled
to true), applications will never receive this event as in some other controls.
Click is one of many button events, for a full list see here.
A button can raise a click event in the code-behind. Alternatively you can bind an instance of ICommand
to the command property. The bound command will then be executed whenever the button is clicked.
For guidance on how to bind to a command, see here.
Useful Properties
You will probably use these properties most often:
Property | Description |
---|---|
ClickMode | Describes how the button should react to clicks. |
Command | An instance of ICommand to be invoked when the button is clicked. |
Example
This example shows a simple button and a C# code-behind click event handler.
<StackPanel Margin="20">
<Button Click="ClickHandler">Press Me!</Button>
<TextBlock Margin="0 10" x:Name="message">Ready...</TextBlock>
</StackPanel>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
public void ClickHandler(object sender, RoutedEventArgs args)
{
message.Text = "Button clicked!";
}
}
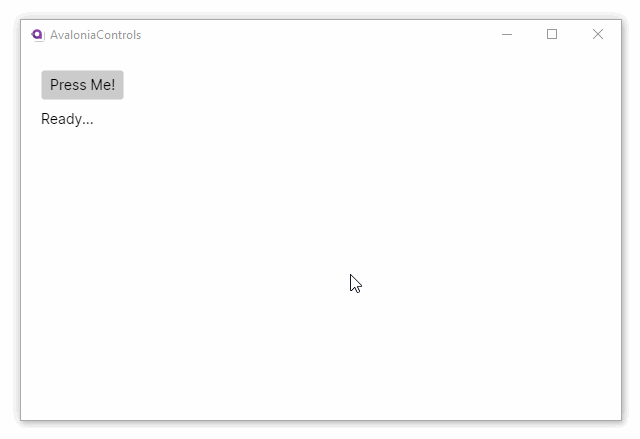
More Information
For the complete API documentation about this control, see here.
View the source code on GitHub Button.cs