How To Use Live Preview
This guide will show you how to use the live preview feature of the Avalonia UI extensions for Visual Studio and ReSharper.
The Avalonia for Visual Studio extension includes a XAML designer which can be used to show a live preview of the XAML as you are writing it. With the Avalonia for Visual Studio extension installed, double click on an Avalonia XAML file to open it.
For Visual Studio and ReSharper users, ReSharper 2020.3 introduces built-in code analysis, code completion, navigation, and find usages.
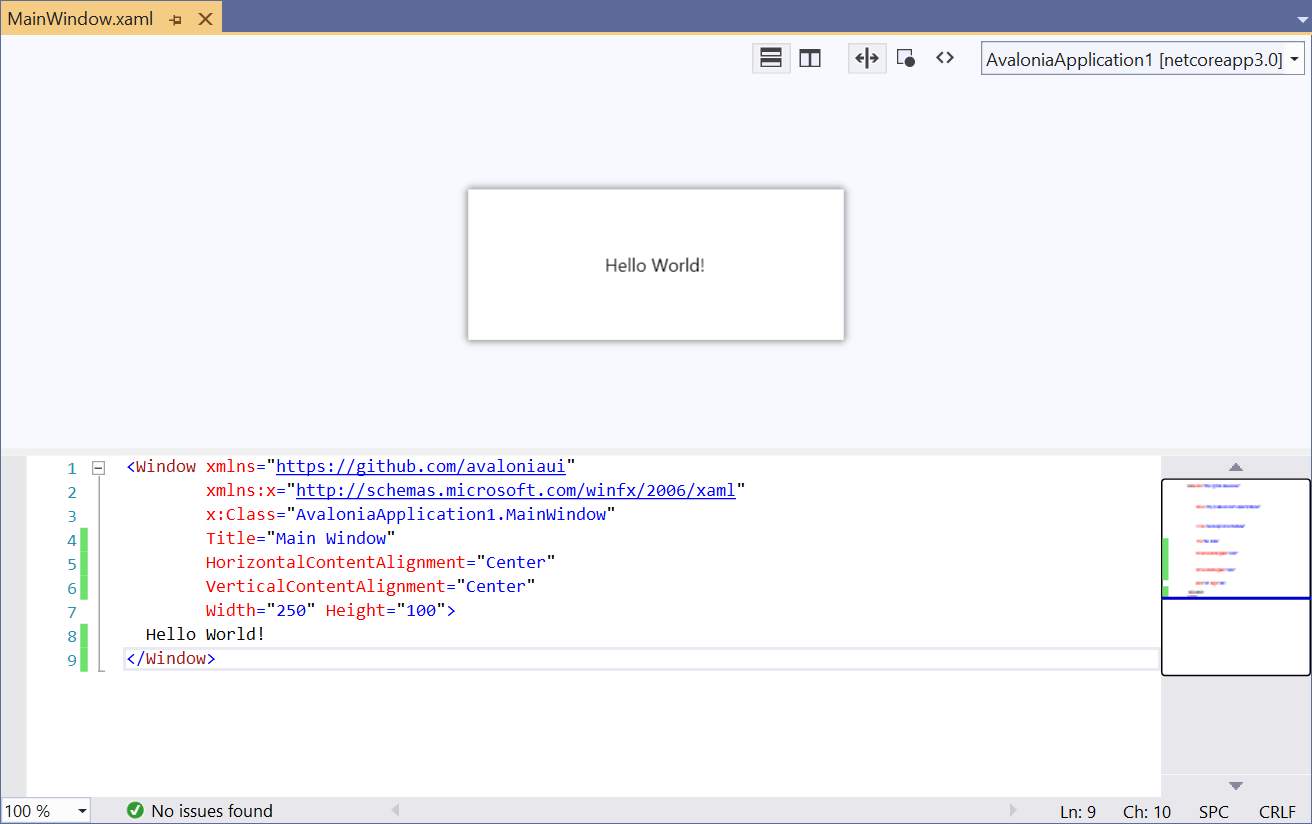
If your XAML is in a library, Avalonia UI needs an executable application in order to be able to preview it. Select an executable project from the dropdown on the top right of the designer. Once your project is built, editing the XAML in the editor will cause the preview to update automatically.
In some cases, due to bugs/limitations in Visual Studio, the Avalonia XAML designer is not shown and instead the WPF designer gets shown. If your XAML file is showing a lot of errors, try right-clicking the file then selecting "Open With..." → "Avalonia XAML Editor".
Design-Time Properties
There are a number of properties that can be applied to your controls which will take effect only at design-time. To use these you must add a namespace to your XAML file:
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
With the namespace added, the following design-time properties become available:
d:DesignWidth and d:DesignHeight
The d:DesignWidth
and d:DesignHeight
properties apply a width and height to the control being previewed.
<Window xmlns="https://github.com/avaloniaui"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
d:DesignWidth="800" d:DesignHeight="450"
x:Class="AvaloniaApplication1.MainWindow">
Welcome to Avalonia!
</Window>
d:DataContext
The d:DataContext
property applies a DataContext
only at design-time. It is recommended that you use this property in conjunction with the {x:Static}
directive to reference a static property in one of your assemblies:
<Window xmlns="https://github.com/avaloniaui"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:dd="clr-namespace:My.Namespace;assembly=MyAssembly"
d:DataContext="{x:Static dd:DesignData.ExampleViewModel}"
x:Class="AvaloniaApplication1.MainWindow">
Welcome to Avalonia!
</Window>
namespace My.Namespace
{
public static class DesignData
{
public static MyViewModel ExampleViewModel { get; } = new MyViewModel
{
// View Model initialization here.
};
}
}
Design.DataContext
Alternatively you can use Design.DataContext
attached property. As well as Design.Width
and Design.Height
.
<Window xmlns="https://github.com/avaloniaui"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:dd="clr-namespace:My.Namespace;assembly=MyAssembly"
x:Class="AvaloniaApplication1.MainWindow"
Design.Width="100">
<Design.DataContext>
<dd:MyViewModel />
</Design.DataContext>
Welcome to Avalonia!
</Window>
Setting the Design-Time DataContext in Code
Setting the design-time DataContext
in XAML by one of the methods shown above is only possible if the view model has a parameterless constructor. Yet view model constructors with parameters are required with typical dependency injection patterns. One option is to use a special design-time view model with a parameterless constructor. However, a design-time DataContext
that references a view model constructor with parameters may be set in code with the Design.SetDataContext
method.
public MainWindow()
{
// Prevent the previewer's DataContext from being set when the application is run.
if (Design.IsDesignMode)
{
// This can be before or after InitializeComponent.
Design.SetDataContext(this, new MainWindowViewModel(new DialogService()));
}
InitializeComponent();
}
Diagnosing Errors
If you're having problems, try enabling verbose logging. To do this in Visual Studio, follow this procedure:
- Click Options... on the Tools menu
- Click Avalonia XAML Editor in the list
- Click Verbose in the Minimum Log Verbosity drop-down.
Logs will now be displayed in the Visual Studio Output window.
Select Avalonia Diagnostics from the drop-down at the top of this window.